https://nnuoyos.tistory.com/302
저번 포스팅에 이어서
react redux 공식 문서에 나와있는 대로 사용해준다
dispatch 함수의 액션은 객체 형태로 되어 있다
dispatch({type: , payload: })
반드시 타입이라는 key가 있어야 하고
액션 안에는 payload 가 있는데 이건 옵션처럼 사용할 수 있다
useDispatch를 통해 액션을 던져준 것이다
App.js
import { useState } from 'react';
import './App.css';
import { useDispatch } from 'react-redux';
function App() {
const [counter, setCounter] = useState(0);
const dispatch = useDispatch()
const increase = () => {
dispatch({type: "INCREMENT"})
setCounter(counter+1);
};
return (
<div>
<h1>{counter}</h1>
<button onClick={increase}>증가</button>
</div>
);
}
export default App;
reducer.js
let initialState = {
count : 0,
}
function reducer(state=initialState, action){
console.log("액션 확인!", action)
}
export default reducer;
지정해놓은 타입이 나온다
let initialState = {
count : 0,
}
function reducer(state=initialState, action){
console.log("액션 확인!", action);
if(action.type == "INCREMENT"){
return {...state, count: state.count + 1}
}
return {...state}
}
export default reducer;
액션 타입이 INCREMENT 이면 객체를 리턴한다
리듀서는 스토어를 바꾸는 역할을 하는데 규칙을 바꾼다 (리턴 값으로 바꿔주게 된다)
리듀서는 항상 스테이트 값을 바꿔주게 된다
return {...state, count: state.count + 1}
state가 여러개 있을 수도 있고, 내가 모르는 스테이트들은 그대로 유지하되,
count+1 값만 count 스테이트에 변경한다는 뜻
스프레드 연산(...)으로 기존의 객체 내용을 복사하고, 새로운 객체에 전달하는 형식으로 사용해야 한다
다른 컴포넌트들에게 값을 전달
스토어는 리턴 값을 무조건 받아야하기 때문에 if 에 해당하지 않더라도 리턴값으로 ...state 를 반환해야 한다
리듀서가 행동지침을 들고 있기 때문!
회사마다 사용하는 리듀서의 행동지침이 다르기 때문에
if / switch 등등으로 사용할 수 있다
/* switch문으로 행동지침을 작성한다면 */
switch(action.type){
case "INCREMENT":
return {...state, count: state.count + 1}
default: return {...state}
}
App.js
/* import { useState } from 'react'; */
import './App.css';
import { useDispatch, useSelector } from 'react-redux';
function App() {
/* const [counter, setCounter] = useState(0); */
const dispatch = useDispatch()
const count = useSelector((state) => state.count);
const increase = () => {
dispatch({type: "INCREMENT"})
/* setCounter(counter+1); */
};
return (
<div>
<h1>{count}</h1>
<button onClick={increase}>증가</button>
</div>
);
}
export default App;
리덕스를 사용할 것이기 때문에 기존에 사용한 useState는 지워준다
useSelector 는 함수를 매개변수로 받아온다
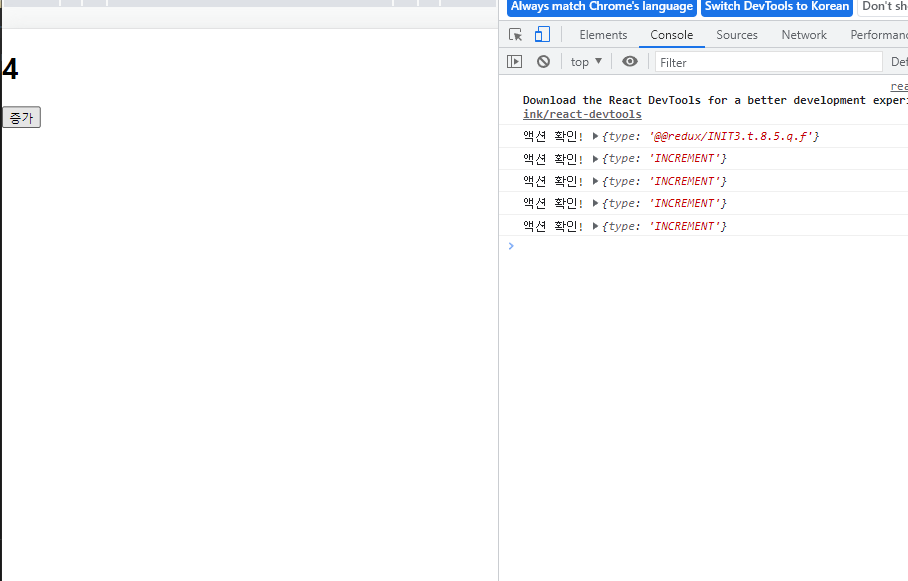
이제 useState를 사용하지 않고도 실행이 가능하다
새 컴포넌트를 만들어준다
Box 에서도 숫자를 보여주도록 하기
스테이트 값을 가져오고 싶으면 useSelctor 를 사용한다
Box.js
import React from 'react'
import { useSelector } from 'react-redux'
const Box = () => {
let count = useSelector((state) => state.count)
return (
<div>This is Box{count}</div>
)
}
export default Box
이제 다시 Box 내부에 새로운 컴포넌트를 만들어보자
GrandSonBox.js
import React from 'react'
import { useSelector } from 'react-redux'
const GrandSonBox = () => {
let count = useSelector((state)=>state.count)
return (
<div>GrandSonBox{count}</div>
)
}
export default GrandSonBox
Box.js
import React from 'react'
import { useSelector } from 'react-redux'
import GrandSonBox from './GrandSonBox'
const Box = () => {
let count = useSelector((state) => state.count)
return (
<div>
This is Box{count}
<GrandSonBox/>
</div>
)
}
export default Box
이렇게 되면 props로 하나씩 던져주지 않아도 사용 가능하다
감소되는 버튼도 만들기
App.js
/* import { useState } from 'react'; */
import './App.css';
import { useDispatch, useSelector } from 'react-redux';
import Box from "./component/Box"
function App() {
/* const [counter, setCounter] = useState(0); */
const dispatch = useDispatch()
const count = useSelector((state) => state.count);
const increase = () => {
dispatch({type: "INCREMENT"})
}
const decrease = () => {
dispatch({type: "DECREMENT"})
}
return (
<div>
<h1>{count}</h1>
<button onClick={increase}>증가</button>
<button onClick={decrease}>감소</button>
<Box/>
</div>
);
}
export default App
reducer.js
let initialState = {
count : 0,
}
function reducer(state=initialState, action){
console.log("액션 확인!", action);
/* if(action.type == "INCREMENT"){
return {...state, count: state.count + 1}
}
return {...state} */
/* switch문으로 행동지침을 작성한다면 */
switch(action.type){
case "INCREMENT":
return {...state, count: state.count + 1}
case "DECREMENT":
return {...state, count: state.count - 1}
default: return {...state}
}
}
export default reducer;
'Dev. > React' 카테고리의 다른 글
Redux (0) | 2022.09.30 |
---|---|
React :: 배포하기 (0) | 2022.09.20 |
React :: shopping site 만들기 05 (0) | 2022.09.20 |
React :: shopping site 만들기 04 (0) | 2022.09.19 |
React :: shopping site 만들기 03 (1) | 2022.09.16 |
댓글